Integrate Checkout Page
The Checkout Page is the most common method used to integrate FasterPay as a payment option. Once your customer is ready to pay, FasterPay will take care of the payment, notify your system about the payment and return the customer back to your Thank You page.
Prerequisites
To integrate the Checkout Page you will need your API keys which you can find in your FasterPay Business account. If you don’t have a FasterPay Business account yet, you can sign up for one.
You can start testing the API and follow the steps below using the Test Mode and Go Live after.
How does the Checkout Page integration work?
- The customer clicks on the Pay Button on your website and is redirected to FasterPay checkout page.
- The customer makes a payment and FasterPay sends a Pingback to your servers.
- FasterPay redirects the customer to your Thank You page.
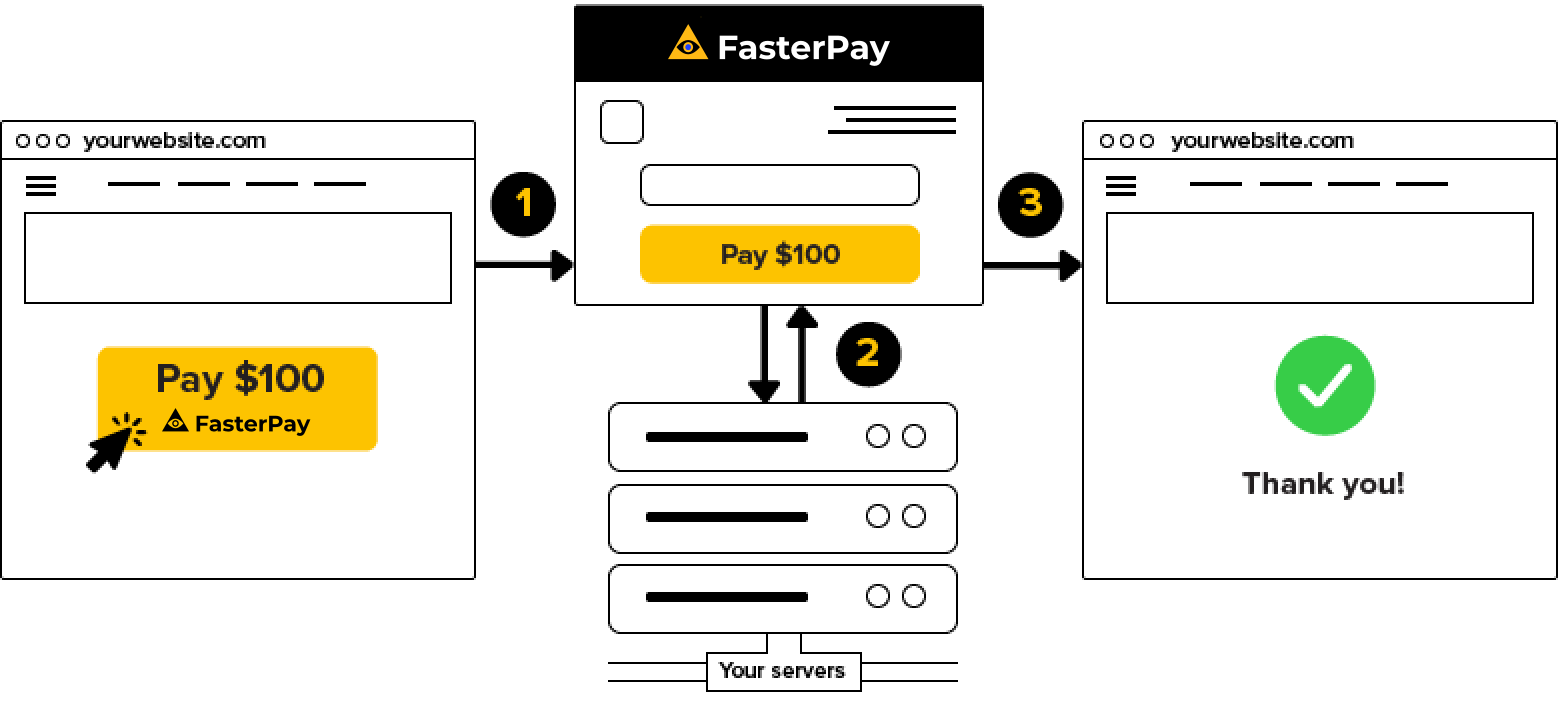
To integrate the Checkout Page follow the steps below:
Step 1: Redirect your customer to the Checkout Page
Once your customer wants to pay, redirect them to FasterPay Checkout Page using the code below:
<?phprequire_once('path/to/fasterpay-php/lib/autoload.php');
$gateway = new FasterPay\Gateway([
'publicKey' => '<your-public-key>',
'privateKey' => '<your-private-key>',
'isTest' => 1,
]);
$form = $gateway->paymentForm()->buildForm(
[
'description' => 'Test order',
'amount' => '10',
'currency' => 'USD',
'merchant_order_id' => time(),
'success_url' => 'https://yourcompanywebsite.com/success',
'pingback_url' => 'https://yourcompanywebsite.com/pingback',
'sign_version' => 'v2' // to use version 1 please skip this param or set it 'v1'
],
[
'autoSubmit' => false,
'hidePayButton' => false
]
);
echo $form;
from fasterpay.gateway import Gateway
import random
if __name__ == "__main__":
gateway = Gateway("<your private key>", "<your public key>")
parameters = {
"payload": {
"description": "Golden Ticket",
"amount": "0.01",
"currency": "EUR",
"merchant_order_id": random.randint(1000, 9999),
"success_url": "http://localhost:12345/success.php",
},
"auto_submit_form": True
}
paymentForm = gateway.payment_form().build_form(parameters)
print paymentForm
const fasterpay = require('fasterpay-node');
let gateway = new fasterpay.Gateway({
publicKey: '<your public key>',
privateKey: '<your private key>',
isTest: 1 // Use 1 for Test Method
});
app.get('/one-time', (req, res) => {
let paymentForm = gateway.PaymentForm().buildForm({
'description': 'Test order',
'amount': '10',
'currency': 'USD',
'merchant_order_id': new Date().getTime().toString(),
'sign_version': 'v2',
'success_url': baseurl + '/success',
'pingback_url': baseurl + '/pingback'
},{
'autoSubmit': false,
'hidePayButton': false,
});
res.send(paymentForm);
});
public class TransactionServlet extends HttpServlet {
private Gateway gateway = Gateway.builder()
.publicApi("<your public key>")
.privateApi("<your private key>")
.build();
@Override
protected void doPost(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
PrintWriter writer = resp.getWriter();
Form form = gateway.paymentForm()
.amount("5.00")
.currency("USD")
.description("Golden ticket")
.merchantOrderId(UUID.randomUUID().toString())
.sign_version(SignVersion.VERSION_2)
.isAutoSubmit(true);
writer.println("<html><body>");
writer.println(form.build());
writer.println("</body></html>");
}
}
class MainActivity : AppCompatActivity() {
val fasterPay: FasterPay by lazy {
FasterPay("<your public key>", true)
}
private fun requestPayment() {
val form = gateWay.form()
.amount("0.5")
.currency("USD")
.description("Golden Ticket")
.merchantOrderId(UUID.randomUUID().toString())
.successUrl("<your definition url>")
startActivity(gateWay.prepareCheckout(this, form))
}
}
curl https://pay.fasterpay.com/payment/form \
-d "api_key: [YOUR_PUBLIC_KEY]" \
-d "merchant_order_id=w2183261236" \
-d "amount=9.99" \
-d "currency=USD" \
-d "email=user@host.com" \
-d "description=Golden Ticket" \
-d "hash=<hash generated using the combination of post params and merchant private key>"
You can find more information on the API parameters in the Checkout Page API.
Step 2: Process the Pingback
After the customer makes a payment, FasterPay will send a Pingback to your servers notifying you about the payment. You can use the code below to validate the Pingback, track the payment in your server and deliver the product:
<?phprequire_once('path/to/fasterpay-php/lib/autoload.php');
$gateway = new FasterPay\Gateway([
'publicKey' => '<your-public-key>',
'privateKey' => '<your-private-key>',
'isTest' => 1,
]);
$signVersion = FasterPay\Services\Signature::SIGN_VERSION_1;
if (!empty($_SERVER['HTTP_X_FASTERPAY_SIGNATURE_VERSION'])) {
$signVersion = $_SERVER['HTTP_X_FASTERPAY_SIGNATURE_VERSION'];
}
$pingbackData = null;
$validationParams = [];
switch ($signVersion) {
case FasterPay\Services\Signature::SIGN_VERSION_1:
$validationParams = ["apiKey" => $_SERVER["HTTP_X_APIKEY"]];
$pingbackData = $_REQUEST;
break;
case FasterPay\Services\Signature::SIGN_VERSION_2:
$validationParams = [
'pingbackData' => file_get_contents('php://input'),
'signVersion' => $signVersion,
'signature' => $_SERVER["HTTP_X_FASTERPAY_SIGNATURE"],
];
$pingbackData = json_decode(file_get_contents('php://input'), 1);
break;
default:
exit('NOK');
}
if (empty($pingbackData)) {
exit('NOK');
}
if (!$gateway->pingback()->validate($validationParams)) {
exit('NOK');
}
#TODO: Write your code to deliver contents to the End-User.
echo "OK";
exit();
from flask import request
from fasterpay.gateway import FP_Gateway
gateway = Gateway("<your private key>", "<your public key>")
if gateway.pingback().validate({"apiKey": request.headers.get("X-ApiKey")}) is True:
print "OK"
else:
print "NOK"
const fasterpay = require('fasterpay-node');
let gateway = new fasterpay.Gateway({
publicKey: '<your public key>',
privateKey: '<your private key>',
isTest: 1 // Use 1 for Test Method
});
app.post("/pingback", (req, res) => {
let html = 'NOK';
if(gateway.Pingback().validate(req)){
html = 'OK';
}
res.send(html);
});
import spark.Service;
public class PingBackRoute implements Routes {
private PingBack pingBack = new PingBack("<your private key");
@Override
public void define(Service service) {
this.service = service;
definePingbackRoutes();
}
@POST
public void definePingbackRoutes() {
service.post(BASE_PATH, ((request, response) -> {
boolean isValid = pingBack.validation()
.signVersion(Optional.ofNullable(request.headers(PingBack.X_FASTERPAY_SIGNATURE_VERSION)))
.apiKey(Optional.ofNullable(request.headers(PingBack.X_API_KEY)))
.signature(Optional.ofNullable(request.headers(PingBack.X_FASTERPAY_SIGNATURE)))
.pingBackData(Optional.of(request.body()))
.execute();
if (isValid) {
//process further with pingBack
response.status(HttpStatus.OK_200);
return response;
} else {
//process with invalid pingBack
throw halt(HttpStatus.NOT_IMPLEMENTED_501);
}
}));
}
}
curl --header "Content-Type: application/json" \
--request POST \
--data '{ \
"event":"payment", \
"payment_order":{ \
"id":273281973, \
"merchant_order_id":"w2981398127", \
"payment_system":1, \
"status":"successful", \
"paid_amount":46.049999999999997, \
"paid_currency":"EUR", \
"merchant_net_revenue":42.227200000000003, \
"merchant_rolling_reserve":2.3025000000000002, \
"fees":1.5203, \
"date":{ \
"date":"2018-11-30 23:59:10.000000", \
"timezone_type":3, \
"timezone":"UTC" \
} \
}, \
"user":{ \
"firstname":"John", \
"lastname":"Doe", \
"username":"John-Doe@my.passport.io", \
"country":"US", \
"email":"johndoe@example.com" \
}, \
"with_risk_check":false, \
}'
https://your-pingback-url.com/pingback.php
FasterPay expects the following response: Response Status: 200 Response Body: ok
In case a different response is received, FasterPay will resend Pingbacks every 30 minutes for up to 10 times. You can also accept a range of Pingback types, such as refunds and chargebacks. More about Pingbacks.
Step 3: Show your Thank You page
Once the user makes a payment, they will be redirected to the success_url page that you specify during step.1. You can show your “Thank You” message on this screen.
Step 4: Integrate Delivery Confirmation API
For every product purchased by an end-user, merchants that don’t instantly deliver the products are required to implement FasterPay Delivery Confirmation API and communicate the delivery status of the product / service.
This information is important for our customer service teams in order to investigate disputes raised by end-users in a better way.
Requirements for E-commerce businesses
As a general rule, card schemes (e.g. Visa, MasterCard) do not allow capturing a transaction until goods have been shipped or services rendered.
When a user makes a purchase on your E-commerce store, FasterPay only authorizes the transaction. You are expected to ship the goods within 7 days of handling time. If an order is not shipped within 7 days - the transaction is automatically voided and the funds are returned to the user.
A proof of shipping should be communicated to FasterPay automatically via Delivery Confirmation API with status=order_shipped along with tracking ID, tracking link and carrier name. Upon receiving the confirmation, FasterPay captures the payment and the funds move to your Pending Balance.
Instructions can be found in the APIs section here: Delivery Confirmation API
If you are using FasterPay’s plugins in your E-Commerce stores, please refer to respective plugin documentation on configuring Delivery Confirmation.
Step 5: Test
Once you have completed implementation, go ahead and test it using the Test Credit Card numbers found in FasterPay’s Test Mode. You can turn on test mode by clicking the toggle in the header.
Step 6: Go Live
Once you’re done, simply fill out your Business Profile and Go Live!